Learning ColdFusion 9: When Does An ORM-Enabled Object Get Persisted
From what I have seen so far, the ColdFusion 9 ORM / Hibernate integration is very interesting. During my exploration of this feature, however, I ran into a persistence behavior that definitely confused me at first. From my initial read of the ORM documentation, I had thought that all matters of persistence were controlled by the EntitySave() method. As it turns out, though, this is not true - once an object has been persisted, all future updates to that object are automatically persisted after the Hibernate session ends (at request / transaction termination) or when the ORM updates are flushed manually.
On further reading of the various ORM blog posts out there, it seems that this is the correct behavior. But, since it confused me, I figured I would share my experience with others who might, at first, not understand what's going on. To test the ORM persistence behavior, I set up three simple ColdFusion pages that all interact with a single entity in a different way.
New ORM-Enabled Object
The first page that I wrote simply created a new object and stored some default data:
<h1> | |
ColdFusion 9 ORM: New Object | |
</h1> | |
<!--- | |
Create a new Task object, passing some default values | |
to the object constructor. | |
---> | |
<cfset task = new com.Task( | |
"Ask Joanna to a movie.", | |
"Gather the courage and just ask her out!" | |
) /> | |
<!--- Output the new, non-persited task. ---> | |
<cfdump | |
var="#task#" | |
label="New Task" | |
/> | |
<!--- | |
Check to see if are exiting out of the page. This is | |
here to see if new instances are saved without explicitly | |
calling entitySave(). | |
---> | |
<cfif isNull( url.save )> | |
<cfexit /> | |
</cfif> | |
<br /> | |
<!--- Save the task. ---> | |
<cfset entitySave( task ) /> | |
<!--- Output the updated, persisted task. ---> | |
<cfdump | |
var="#task#" | |
label="Saved Task." | |
/> |
As you can see, the code above can run in two different modes. If I run it without any URL flags, the new Task.cfc instance will be created but the template will exit without EntitySave() getting called. I did this to confirm that new objects would not be persisted without explicit ORM interaction. And, in fact, if I run this code as above, no record gets stored in the database.
If, however, I run the code with the URL parameter, "save," then EntitySave() does get executed, the CFC gets persisted, and a new record is stored in the database. This confirms the documented information that a new object will not be persisted until EntitySave() is explicitly called on it.
Existing ORM-Enabled Object
The second page that I wrote simply loads an existing object based on its primary key and outputs it:
<h1> | |
ColdFusion 9 ORM: Existing Object | |
</h1> | |
<!--- | |
Load an existing task based on it's primary key. | |
Unlike entityLoad(), the entityLoadByPK() method | |
loads by primary key and returns a given instance, | |
not an array of matching instances. | |
NOTE: If no matching task is found, a null value | |
is returned. | |
---> | |
<cfset task = entityLoadByPK( "Task", 1 ) /> | |
<!--- Output the existing task information. ---> | |
<cfdump | |
var="#task#" | |
label="Existing Task" | |
/> |
This page is just here to help test what data is available after the following page has been run.
Updating An Existing ORM-Enabled Object
The third and final page that I wrote loads an existing ORM-enabled object and updates one of its properties:
<h1> | |
ColdFusion 9 ORM: Update Object | |
</h1> | |
<!--- | |
Load an existing task based on it's primary key. | |
Unlike entityLoad(), the entityLoadByPK() method | |
loads by primary key and returns a given instance, | |
not an array of matching instances. | |
NOTE: If no matching task is found, a null value | |
is returned. | |
---> | |
<cfset task = entityLoadByPK( "Task", 1 ) /> | |
<!--- Flag this task as having been completed. ---> | |
<cfset task.setIsComplete( true ) /> | |
<!--- Output the existing task information. ---> | |
<cfdump | |
var="#task#" | |
label="Existing Task" | |
/> |
What you'll notice here is that while I am updating the IsComplete property of the Task.cfc instance, I am at no time executing an EntitySave() call. But, when I jump back over to the "Existing" ORM-Enabled object page, I get this output:
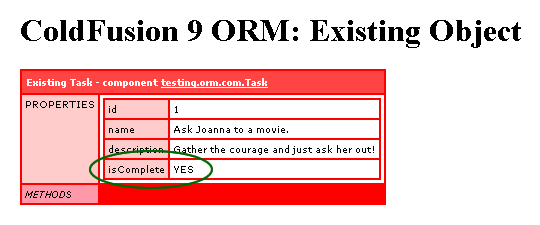
... and when I look at the database, I get these records:
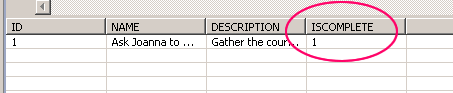
As you can see, even without calling EntitySave(), updates to an already-persisted object are automatically persisted at the end of the Hibernate session (defined implicitly in this case as the end of the page request).
When I first read through the ORM documentation, it gave me the impression that all ORM-based persistence to the database needed to be done through explicit calls to the EntitySave() method. In fact, on the "Perform create, read, update, delete operations on ORM Objects" page of the CF9 Developer's Guide, it explains object updates as:
The method to update an object is the same as saving an object. Load the object that needs to be updated, make updates, and save the object. For example:
<cfset artist1 = EntityLoad("Artist", 1, true)>
<cfset artist1.setFirstName("Garcia")>
<cfset EntitySave(artist1)>
I think from something like this, it's easy to see where I would get my initial impressions. That's why the behavior I was seeing was so confusing. As it turns out, however, once an object has been persisted, any further updates to it will be automatically persisted at the end of the associated Hibernate session.
Want to use code from this post? Check out the license.
Reader Comments
It should also be noted Ben - to update an object, you actually don't have to call EntitySave() at all!
Have a look at my examples from my DevNet article:
http://www.compoundtheory.com/?action=displayPost&ID=417
In the update example, we don't even called EntitySave().
This is because the Hibernate Session is already aware of the object, and will track any changes that are made to the object.
Oh wait.. you knew that. I didn't read your post properly.
Oops.
Ignore me!
* crawls under rock *
This would make for a nice demo at the next NYCFUG.
Would this work equally on any RDBMS?
MSSQL and MySQL don't require any type of COMMIT command be issued before a row is officially updated, where Oracle does (or at least it did when I last worked with it).
Will CF9's ORM implementation handle this transparently?
@Mark,
No worries :)
@David,
Yeah, agreed. Perhaps we'll do some CF9-specific features in the upcoming months. That would be awesome.
@Claude,
As far as I know, this should all be handled transparently. Of course, I'm very new to this, so that is just my *assumption*.
I can say that even the first ColdFusion page you presented had helped me in my work! I am not talking about other pages that are great
This new ORM stuff is really very cool, this is what I think I consider the 'killer feature' in CF9 which will coax me into upgrading, really enjoying watching you guys explore it.
I wonder, has anyone yet done any performance testing on persisting / retrieving large record sets using the ORM features? I'd be keen to know how it shapes up compared to traditional methods, would give a good insight into those planning to use it in high-demand applications.
Rob
@Claude: Both MySQL, MSSQL and Oracle can be configured (and are be default ?) to 'auto commit' without an explicit 'COMMIT;' statement (in it's own CFQUERY) as far as I know.
Certainly last time I used Oracle I didn't need to issue one.
Whoa, this is actually a big deal when considering validation approaches. I had played around with CF9's ORM capabilities awhile ago and did not notice this behaviour. Do you know, is there a way to change this behaviour, so that an object is only saved when EntitySave() is called? Perhaps something one does with the object, or a hibernate configuration setting?
@Bob,
It's funny you mentioned that because when I noticed this behavior, that was the very first thing that popped into my mind. I have seen *many* people populate a CFC just to validate it; it appears that that mentality can no long exist? I'll wait for some more ORM-experts to drop in on this, but I had the same reaction.
Hi Ben, that is a great point that Bob raised about letting an entity validate itself. I had a look into and my response was too long to add here so I posted it here:
http://www.aliaspooryorik.com/blog/index.cfm/e/posts.details/post/cf9-orm-clearing-the-session-cache-227