ColdFusion Arguments Object Can Act As Ghetto Pass-By-Reference Array
As you may or may not know, the ARGUMENTS object that holds the parameters passed to a ColdFusion function can be accessed as either a key-value structure or as an indexed array:
<cffunction | |
name="Massage" | |
access="public"> | |
<!--- Define arguments. ---> | |
<cfargument name="Girl" type="any" required="true" /> | |
<!--- Get girl via arguments STRUCT. ---> | |
<cfset ARGUMENTS.Girl /> | |
<!--- Get girl via arguments ARRAY. ---> | |
<cfset ARGUMENTS[ 1 ] /> | |
<cfreturn /> | |
</cffunction> |
Notice that the "Girl" argument can be accessed as a key or an index. This got me thinking about the ARGUMENTS object. What is it? How does it act? Well, let's start with what it is; when you grab it's underlying Java class and the super class chain, you get this:
- coldfusion.runtime.ArgumentCollection
- coldfusion.util.FastHashtable
- java.util.Hashtable
It's some sort of ArgumentCollection that extends the HashTable (think ColdFusion Struct). Now, as you know, Structures in ColdFusion are passed by reference, not by value. But, they can also be used as Arrays. Does this mean that we could potentially use the ARGUMENTS collection as a pass-by-reference array in ColdFusion?
Before even testing this, we have to figure out how to get an ARGUMENTS collection. You can try to create it using CreateObject(), but you will find that it take two parameters. There are two constructors listed and I could not figure out what either of them were supposed to take.
The easiest way to get an empty ARGUMENTS object is just have an intermediary ColdFusion function return it's own copy:
<!--- | |
This function does nothing but reflect it's own ARGUMENTS object. | |
This will allow us to create empty ARGUMENTS objects without | |
having to know about initialization parameters. | |
---> | |
<cffunction | |
name="GetArguments" | |
access="public" | |
returntype="any" | |
output="false" | |
hint="Returns the arguments object."> | |
<cfreturn ARGUMENTS /> | |
</cffunction> |
Ok, now once we have a way to get a new ARGUMENTS object, we can start to test the functionality. I am guessing that access methods are going to be safer than modification methods, so let's test those first:
<!--- Get an array with defaulted values. ---> | |
<cfset arrArguments = GetArguments( 1, 2, 3, 4, 5 ) /> |
Notice that since our GetArguments() method just returns the array, it allows us to set default array values. This is what this object looks like:
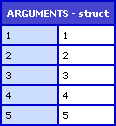
Before we go any further, we have to check to see if this object IS even passed by reference. To test this, let's create a function that modifies a passed-in array but does NOT return it:
<cffunction | |
name="AlterArray" | |
access="public" | |
returntype="void" | |
output="false" | |
hint="Alter an array by setting first index."> | |
<!--- Alter first index of first argument. ---> | |
<cfset ARGUMENTS[ 1 ][ 1 ] = "999" /> | |
<!--- Return out (but do NOT return array). ---> | |
<cfreturn /> | |
</cffunction> |
Passing in our array to this function:
<!--- Try to alter array by passing by reference. ---> | |
<cfset AlterArray( arrArguments ) /> | |
<!--- Dump out original array. ---> | |
<cfdump | |
var="#arrArguments#" | |
label="ARGUMENTS" | |
/> |
This gives us the following array object:
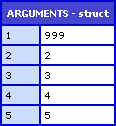
Ok, so we see that the object is in fact passed by reference. Now, let's test the access methods:
ArrayAvg() : #ArrayAvg( arrArguments )#<br /> | |
ArrayIsEmpty() : #ArrayIsEmpty( arrArguments )#<br /> | |
ArrayLen() : #ArrayLen( arrArguments )#<br /> | |
ArrayMax() : #ArrayMax( arrArguments )#<br /> | |
ArrayMin() : #ArrayMin( arrArguments )#<br /> | |
ArraySum() : #ArraySum( arrArguments )#<br /> | |
ArrayToList() : #ArrayToList( arrArguments )#<br /> | |
IsArray() : #IsArray( arrArguments)#<br /> |
This gives us the following output:
ArrayAvg() : 3
ArrayIsEmpty() : NO
ArrayLen() : 5
ArrayMax() : 5
ArrayMin() : 1
ArraySum() : 15
ArrayToList() : 1,2,3,4,5
IsArray() : NO
As you can see, everything but the IsArray() method acts just like we would think it would.
I would demonstrate the array modification methods:
ArrayInsertAt() : #ArrayInsertAt( arrArguments, 1, 5 )#<br /> | |
ArrayClear() : #ArrayClear( arrArguments )#<br /> | |
ArrayInsertAt() : #ArrayInsertAt( arrArguments, 1, 5 )#<br /> | |
ArrayPrepend() : #ArrayPrepend( arrArguments, 6 )#<br /> | |
ArrayResize() : #ArrayResize( arrArguments, 2 )#<br /> | |
ArraySet() : #ArraySet( arrArguments, 2, 10, 1000 )#<br /> | |
ArraySort() : #ArraySort( arrArguments, "textnocase" )#<br /> | |
ArraySwap() : #ArraySwap( arrArguments, 1, 2 )#<br /> |
... but, MOST OF THESE CAUSED PROBLEMS! It was actually really disappointing :( So, bottom line is, you can use the ARGUMENTS object as a read-only, pass-by-reference array, but it doesn't quite work. And, if you are gonna use it as a read-only array, you might as well just create a Java ArrayList which is passed by reference anyway and is not as ghetto.
Want to use code from this post? Check out the license.
Reader Comments
This reminds me of a problem I ran into a while back. I was trying to get some code working for grabbing info from some websites. I had to get cookies working properly and the documentation for cfhttp said cookies are returned in an array. I had random problems and I think it worked at one point but in later versions of CF failed. Anyways, it turned out that the variable was actually a struct with numbered keys, not an array, which caused problems of course.
Now thats out of the way... What were you hoping to accomplish with this? Did you have some particular uses in mind? I know I've run into situations where pass by reference could be useful but nothing springs to mind other than maybe putting a reference into a different scope.
Daniel,
I had no goal to accomplish here. I was on my way home the other day and suddenly I realized that I had no idea "what" the ARGUMENTS scope even was. I knew it could be accessed as both an array and as a struct, so, it couldn't be either of them at the same time.
I just wanted to play, see what would happen.
Dear Ben,
Your website is an incredible resource - you really need to get a job at Adobe as a product evangelist! You seem to have much more insight into the language than anybody else I have come across.
I have one *minor* problem - you spell "its" wrongly several times in this posting. "It's" means "it is" or "it has", not "of it".
e.g. "ColdFusion function return it's own copy"
Should read: "ColdFusion function return its own copy".
Thanks again for your blog!
With best wishes,
Mark Winterton
First -- yes, I know this is an old topic. However, I ran into a problem the other day relating to arguments and arrayClear. For example:
It's almost like CF points the variable at a new array, instead of clearing what it is already pointing to. Is this something like what you saw in your research for this? You said "most of these caused problems" but you didn't say what kind of problem you were talking about.
Just curious!
Hi Ben,
On a somewhat related topic, is there an easy way to forward arguments from one function to another? Something like this:
<cffunction name="conditionalForward" ...>
<!-- condition met -->
<cfset variables.obj.anotherFunction( arguments=arguments ) />
</cffunction>