Using The XOR Operator To Assert Connascence Of Existence In ColdFusion
In all my years of programming, I don't think I've ever used the XOR
operator. The XOR
operator—or, "Exclusive OR" operator—is a Boolean operator that results in False if the given operands have the same truthiness; and, True if the given operands have different truthiness:
The same truthiness results in false
:
true XOR true
→false
false XOR false
→false
Mismatched truthiness results in true
:
true XOR false
→true
false XOR true
→true
It's an interesting operator; but, I never had a use-case for it until yesterday. It occurred to me that I could use XOR
to assert the "connascence of existence" of optional method arguments in ColdFusion.
The term "connascence" means to be "born together". The term was first introduced within the context of programming in the book, Fundamentals Of Object-Oriented Design In UML by Meilir Page-Jones. It is meant to describe the relation between two things.
In some ColdFusion code that I'm working on, I have a method that "trues up" a sort
column in a database. Essentially, it looks at the records that are in place and updates the sort
column so that it matches the index of each row (by removing any sparseness between rows). Part of this method call accepts two optional arguments that assert the sort
of a given record:
<cfscript>
private void function trueUpSort(
required numeric projectID,
numeric sectionID, // Optional argument.
numeric sectionSort // Optional argument.
);
</cfscript>
This method, trueUpSort()
, will correct the sort
column of the sections defined within the given projectID
. And—if provided—will ensure that the section with the given sectionID
will be located at the given sectionSort
when all is said and done.
The sectionID
and sectionSort
arguments are both optional; but they aren't independently optional—they have connascence of existence. Meaning, either both of the optional arguments must be provided; or, both of the optional arguments must be omitted. Their existence cannot be mismatched.
Which is exactly what the XOR
operator it testing for. Within the body of this method we can use the XOR
operator to assert that the optional arguments exist in a similar state:
<cfscript>
private void function trueUpSort(
required numeric projectID,
numeric sectionID, // Optional argument.
numeric sectionSort // Optional argument.
) {
// Test for connascence of existence.
if ( isNull( sectionID ) XOR isNull( sectionSort ) ) {
// .... throw() ....
}
}
</cfscript>
If both optional arguments are provided or omitted, this code works fine. However, if only one of the option arguments is defined, this internal assertion will throw an error.
Let's test this ColdFusion code by invoking the method four times with all possible combinations of optional arguments:
<cfscript>
tests = [
{
context: "BOTH of the optional arguments are omitted.",
projectID: 4
},
{
context: "BOTH of the optional arguments are provided.",
projectID: 4,
sectionID: 123,
sectionSort: 9
},
{
context: "Only FIRST optional argument is provided.",
projectID: 4,
sectionID: 123
},
{
context: "Only SECOND optional argument is provided.",
projectID: 4,
sectionSort: 9
}
];
for ( test in tests ) {
try {
trueUpSort( argumentCollection = test );
writeDump([
outcome: "PASS",
test: test
]);
} catch ( any error ) {
writeDump([
outcome: "FAIL",
test: test,
error: error.message
]);
}
}
// ------------------------------------------------------------------------------- //
// ------------------------------------------------------------------------------- //
/**
* I "flatten" the sort property of the sections within the given project. If BOTH a
* section ID and a Sort have been provided, they will be "enforced" as part of the
* flattening process.
*/
private void function trueUpSort(
required numeric projectID,
numeric sectionID,
numeric sectionSort
) {
if ( isNull( sectionID ) XOR isNull( sectionSort ) ) {
throw(
type = "InvalidArguments",
message = "Optional arguments failed connascence of existence.",
detail = "[sectionID] and [sectionSort] must either be both provided to or both omitted from the method invocation."
);
}
// .... rest of method logic ....
}
</cfscript>
If we run this ColdFusion test code, we get the following output:
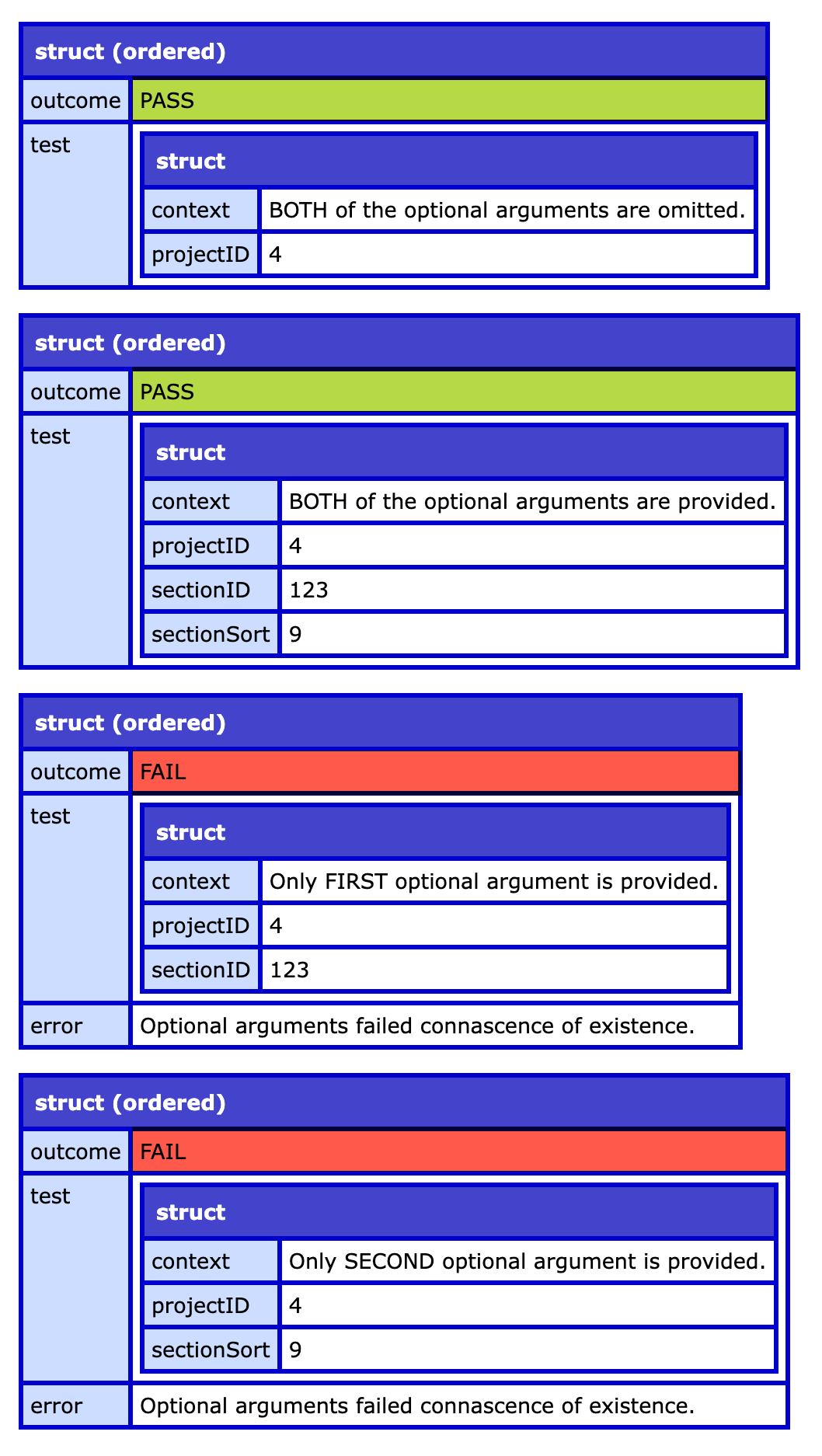
As you can see, the first two method invocations pass. This is for the tests in which both optional arguments are omitted and both optional arguments are provided, respectively. The second two method invocations fail. This if for when only one of the optional arguments is provided.
You could probably argue that this one method should actually be two separate methods: one with and one without the latter two arguments. And, as the ColdFusion application I'm working on evolves, maybe that'll happen. But for the moment, it feels "clean" as one method; and, the XOR
operator gives me peace of mind that I won't accidentally use the optional arguments in an invalid combination.
Want to use code from this post? Check out the license.
Reader Comments
Interesting. This operator has always confused me, probably because I don't have a CS degree. I would have immediately reached for the (AND/OR) operators I know. And that would be much uglier...but maybe more easily understood down the road by someone more simple minded like myself? I'm conflicted because I feel like this falls in the "too cleaver" side of the fence because it's rarely used, but it also falls very neatly on the "code craftsmanship" side of the fence.
@Chris,
So actually someone on LinkedIn suggested that I could achieve the same thing by just doing this:
Since
XOR
is a Boolean operator, the!=
comparison is essentially equivalent. Very clever (and perhaps more clear that way)?But, as far as "too clever" goes, you are likely correct. I had to look up what the
xor
operator did exactly. In this particular case, I would definitely be leaning on thethrow()
to do the heavy lifting of "self documenting" the code.And, as a follow-up here, my original code (the code from which this example was extracted) actually defaults the arguments:
In which case, I could even simplify to:
I love the fact that ColdFusion is 1-based because it means that most things that are
0
are actually "false" (in some respect).@Ben Nadel, yesssss!! both of those examples makes so much more sense to my tiny little brain. Especially the
!=
example. If I used XOR more, it wouldn't be a struggle...but I've never actually used it so if I came across it in a codebase..it would break me (initially)! 😂@Ben Nadel I have also never used
XOR
, but I actually find it very easy to understand.So, am I right in thinking that if one part of the statement is TRUE and the other part is FALSE, then the whole statement is TRUE?
I really hope my understanding is correct, otherwise I will look like a right clown 🤡
If so, I really like this operator and I hope I find a use for it, sometime in the future.
Thanks for taking the time to explain this. 🙂
@Charles,
Exactly right. And, to be clear, the values simply need to be truthy and falsey. Meaning:
( 4 XOR 8 ) == false
Since both
4
and8
are truthy, theXOR
is false despite the fact that the values are not equivalent.@Ben Nadel, So:
As, I understand the only number that is falsey is zero?
Exactly.
@Ben Nadel
So what happens if you have more than two values to test like
Does it just test:
And then:
But which bool value takes precedence?
The truthy or falsey one?
As far as I know, the OR operator, will stop processing at the first truthy evaluation and then uses the truthy evaluation, even if every other evaluation is falsey. So, maybe this is how XOR works as well?
Post A Comment — ❤️ I'd Love To Hear From You! ❤️
Post a Comment →