Using XPath To Select A Node That Does Not Contain Another Node In ColdFusion
Just a quick post on XPath in ColdFusion. This morning, when I was writing about screen-scraping movie showtimes off Fandango.com, I had to use an XPath query that looked for an XML node that did not contain another XML node. In the past, I've blogged about gathering nodes that did not contain a text node (or a given text value); but, I don't think I have ever talked about getting nodes that did not contain a given element node. As such, I wanted to throw together a very brief example of how this is done.
To quickly recap, XPath queries can contain predicates. These are qualitative queries, wrapped in brackets, that filter the given node into or out of the resultant node-set. For example, in the given XPath query:
//a[ @rel = 'home' ]
... we are looking for an "a" element node that has an href attribute that contains the value "home." The predicate, in this case, is the relative query, "[ @rel = 'home' ]". A predicate can contain just about anything so long as that can be boiled down to a truthy / falsey value.
If we want to search for a node that does not contain another node, we can use a negated XPath predicate. Populated node sets are truthy values. As such, if we want to find a node that does not contain a given node, we need to negate the predicate in which the node does contain the given node. In XPath, this negation can be performed by the not() function.
To see this in action, I am going to select from a collection a movies all movies that are not musicals:
<!--- Create the XML document. --->
<cfxml variable="movies">
<movies>
<movie>
<name>Terminator</name>
<genre>
<action />
<adventure />
</genre>
</movie>
<movie>
<name>De-Lovely</name>
<genre>
<musical />
</genre>
</movie>
<movie>
<name>Sex Drive</name>
<genre>
<comedy />
</genre>
</movie>
</movies>
</cfxml>
<!--- Query for all movies that are not musicals. --->
<cfset nonMusicals = xmlSearch(
movies,
"//movie[ not( genre/musical ) ]"
) />
<!--- Output the selected movies. --->
<cfdump
var="#nonMusicals#"
label="Non-musical Movies."
/>
As you can see, our XPath query is using the following search:
//movie[ not( genre/musical ) ]
Within each movie node, we are checking to see if the node contains the ancestor genre, "musical". Of course, since those are the movies that we specifically do not want to select, we then use the not() function to turn any matching musical node set from a truthy value into a false boolean value. This false then filters the given movie out of the resultant node set, returning only movies that do not contain the musical element node.
When we run the above code, we get the following CFDump output:
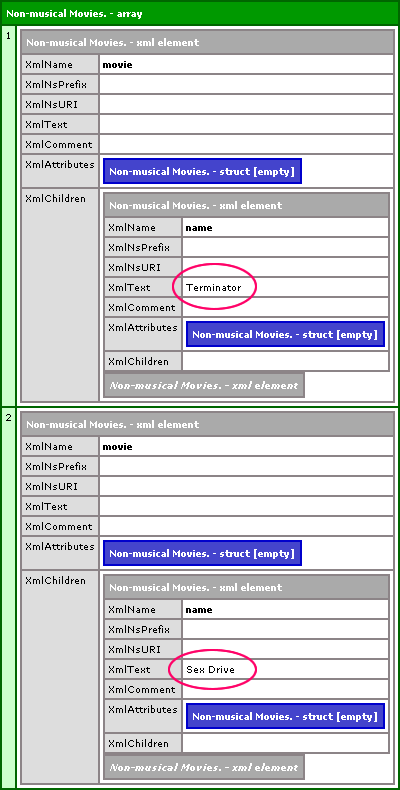
As you can see, De-Lovely, a musical, was not returned in the resultant node set. This is a minor, but powerful feature of XPath.
Want to use code from this post? Check out the license.
Reader Comments
It's good to see there's always something new I can learn about XPath. Good post. Cheers.
--
Adam
@Adam,
No problem my man. XPath is some cool stuff.
hi,
This is the one which help me allot . i am very thankful.