Using style.setProperty() To Keep CSS Property Names Consistent In JavaScript
This morning, while reading an article on theming Angular components by Austin McDaniel, I saw something that I don't think I've ever seen before. Austin was using a .setProperty() method to define an element's CSS properties in JavaScript. Historically, I've mutated the style properties directly using camel-case values. But, one thing that struck me about the .setProperty() approach is that it allows you to use kebab-case, keeping your CSS property names the same in both the CSS and your JavaScript. This is not a critical differentiator; but, it certainly piqued my curiosity (and frustrated me in so much as I didn't know that this method existed). As such, I wanted to give it a quick try.
Run this demo in my JavaScript Demos project on GitHub.
View this code in my JavaScript Demos project on GitHub.
To experiment with the style.setProperty() method in JavaScript, I put together a small demo in which we can alternate the active styles on a given element. One version of the styles will be set using camel-case properties directly on the style object. And, the other version of the styles will be set using kebab-case properties and the setProperty() method:
<!doctype html> | |
<html lang="en"> | |
<head> | |
<meta charset="utf-8" /> | |
<title> | |
Using style.setProperty() To Keep CSS Property Names Consistent In JavaScript | |
</title> | |
<link rel="stylesheet" type="text/css" href="./demo.css"> | |
</head> | |
<body> | |
<h1> | |
Using style.setProperty() To Keep CSS Property Names Consistent In JavaScript | |
</h1> | |
<p> | |
<a class="use-properties">Use Style Properties</a> | |
— | |
<a class="use-set-property">Use Style setProperty()</a> | |
</p> | |
<div class="box"> | |
Goodbye cruel world. | |
</div> | |
<script type="text/javascript"> | |
// Gather DOM references. | |
var useProperties = document.querySelector( ".use-properties" ); | |
var userSetProperty = document.querySelector( ".use-set-property" ); | |
var box = document.querySelector( ".box" ); | |
// Setup our action handlers. | |
useProperties.addEventListener( "click", handleUseProperties, false ); | |
userSetProperty.addEventListener( "click", handleUseSetProperty, false ); | |
// --------------------------------------------------------------------------- // | |
// --------------------------------------------------------------------------- // | |
// I set the box styling using the style object properties. | |
function handleUseProperties( event ) { | |
// Note the use of CAMEL-CASE. | |
box.style.backgroundColor = "#333333"; | |
box.style.border = "2px solid #999999"; | |
box.style.borderRadius = "4px 4px 4px 4px"; | |
box.style.color = "#ffffff"; | |
box.style.cssFloat = "left"; // CAUTION: Using "cssFloat", not "float". | |
// Testing setting and destroying of properties (null destroys). | |
box.style.zIndex = null; | |
box.style.zoom = "1"; | |
} | |
// I set the box styling using the style's setProperty() method. | |
function handleUseSetProperty( event ) { | |
// Note the use of KEBAB-CASE. | |
box.style.setProperty( "background-color", "#fafafa" ); | |
box.style.setProperty( "border", "2px solid #cccccc" ); | |
box.style.setProperty( "border-radius", "4px 4px 4px 4px" ); | |
box.style.setProperty( "color", "#333333" ); | |
box.style.setProperty( "float", "right" ); | |
// Testing setting and destroying of properties (null destroys). | |
box.style.setProperty( "z-index", "1" ); | |
box.style.setProperty( "zoom", null ); | |
} | |
</script> | |
</body> | |
</html> |
As you can see, there's very little going on here. The focal point of this demo is the code that actually defines the properties. When using the .setProperty() method, we can keep our CSS property names the same across our CSS and our JavaScript. This pertains to both the casing of the properties as well as their actual names. Take, for example, the "float" property. When setting it directly, we have to use "cssFloat". But, when setting it using .setProperty(), we can use "float", just like we do in our CSS files.
Now, if we run this code and toggle the styles using .setProperty(), we get the following output:
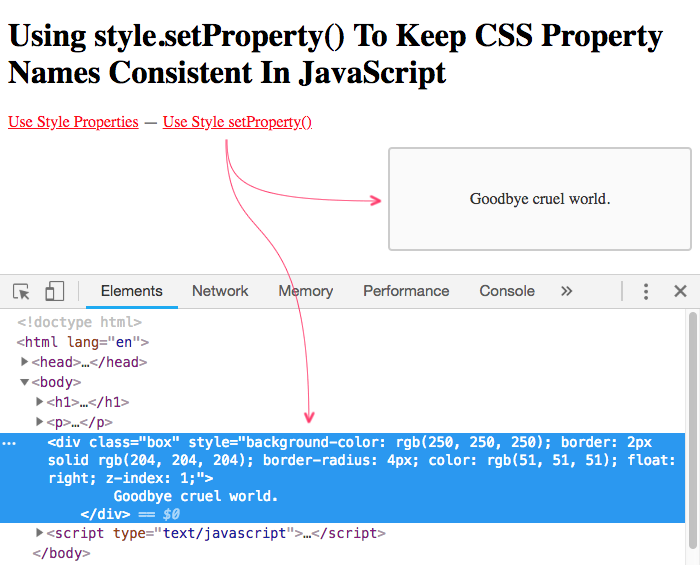
As you can see, the .setProperty() method works perfectly well.
To be clear, I am not recommending that everyone should go out and start using the .setProperty() method instead of mutating the style object directly. But, I am pointing out that this method exists; and, that the consistency it provides does have a certain amount of gravity to it. If nothing else, it's just another tool in the old tool-belt. My mental model is now one step closer to being complete.
Want to use code from this post? Check out the license.
Reader Comments
@All,
So, from what I've been reading, it looks like
.setProperty()
and its sibling.getPropertyValue()
may be the only way to programmatically set and get CSS custom properties (ie, variables). Not entirely sure that's an accurate statement; but, everything that I am reading seems to use these methods for CSS variables.