Translating Global jQuery Event Coordinates To A Local Context
When you use jQuery to capture mouse events (mousedown, mouseup, click, etc.), the jQuery Event object contains the X and Y coordinates of the mouse position at the time the event was triggered. These coordinates, while somewhat different in each browser, have been standardized by jQuery to be available in the pageX and pageY properties. No matter what the target of the event is, these properties are relative to the left and top edges of the document, respectively. Often times, we need the ability to translate these document-relative global coordinates to the coordinate system of the local context (ie. the target element).
When translating global event coordinates to the local context grid, all you have to do, in most cases, is find the offset of the local context element and subtract it from the global coordinates:
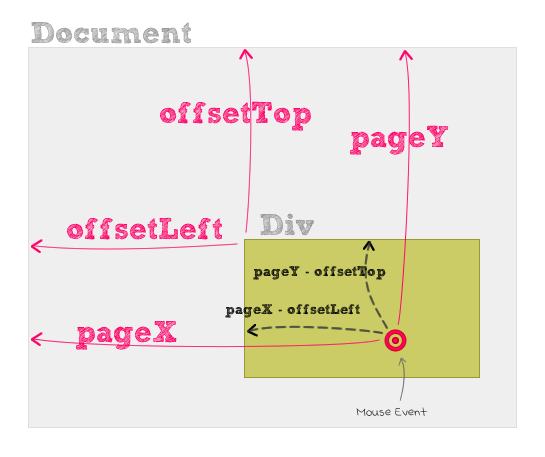
Determining the offset of the local context element would traditionally be something of a nuisance; but, jQuery provides us with the very easy-to-use method: offset(). Calling offset() on a jQuery collection returns the top and left offset (relative to the document) of the first item in the collection. This is not to be confused with the position() method, which returns the top and left offset relative to the offset parent (which is not specifically the document).
Once we have the offset of the local context, we can subtract the "left" from pageX and the "top" from pageY to calculate the local-relevant mouse coordinates. To make this as easy as possible, I have experimented with creating two global jQuery methods: globalToLocal() and localToGlobal(). Both of these require the context element to be passed in; unless, of course, you call them off of the context collection, in which case, the context element will be implied. To see this in action, take a look at the following demo:
<!DOCTYPE HTML> | |
<html> | |
<head> | |
<title>Translating Global jQuery Event Coordinates</title> | |
<style type="text/css"> | |
#target-local, | |
#target-global { | |
background-color: #FAFAFA ; | |
border: 20px solid #CCCCCC ; | |
cursor: pointer ; | |
float: left ; | |
height: 200px ; | |
margin-right: 20px ; | |
padding: 10px 10px 10px 10px ; | |
position: relative ; | |
width: 200px ; | |
} | |
#local { | |
background-color: gold ; | |
border: 1px solid #666666 ; | |
display: none ; | |
padding: 5px 5px 5px 5px ; | |
position: absolute ; | |
white-space: nowrap ; | |
z-index: 100 ; | |
} | |
#global { | |
background-color: #CC0000 ; | |
border: 1px solid #666666 ; | |
color: #FFFFFF ; | |
display: none ; | |
padding: 5px 5px 5px 5px ; | |
position: absolute ; | |
white-space: nowrap ; | |
z-index: 100 ; | |
} | |
</style> | |
<script type="text/javascript" src="jquery-1.4.2.js"></script> | |
<script type="text/javascript"> | |
// I translate the coordiantes from a global context to | |
// a local context. | |
$.globalToLocal = function( context, globalX, globalY ){ | |
// Get the position of the context element. | |
var position = context.offset(); | |
// Return the X/Y in the local context. | |
return({ | |
x: Math.floor( globalX - position.left ), | |
y: Math.floor( globalY - position.top ) | |
}); | |
}; | |
// I translate the coordinates from a local context to | |
// a global context. | |
jQuery.localToGlobal = function( context, localX, localY ){ | |
// Get the position of the context element. | |
var position = context.offset(); | |
// Return the X/Y in the local context. | |
return({ | |
x: Math.floor( localX + position.left ), | |
y: Math.floor( localY + position.top ) | |
}); | |
}; | |
// -------------------------------------------------- // | |
// -------------------------------------------------- // | |
// I am the FN version of the global to local function. | |
$.fn.globalToLocal = function( globalX, globalY ){ | |
return( | |
$.globalToLocal( | |
this.first(), | |
globalX, | |
globalY | |
) | |
); | |
}; | |
// I am the FN version of the local to global function. | |
$.fn.localToGlobal = function( localX, localY ){ | |
return( | |
$.localToGlobal( | |
this.first(), | |
localX, | |
localY | |
) | |
); | |
}; | |
// -------------------------------------------------- // | |
// -------------------------------------------------- // | |
// When the DOM is ready, initialize the scripts. | |
jQuery(function( $ ){ | |
// Get the target divs. | |
var targetLocal = $( "#target-local" ); | |
var targetGlobal = $( "#target-global" ); | |
var local = $( "#local" ); | |
var global = $( "#global" ); | |
// Put the local div inside the target so that its | |
// position will be relative to the target div. | |
targetLocal.append( local ); | |
// Bind to the click event on the target element so | |
// we can trap it and position the elements. | |
targetLocal.click( | |
function( event ){ | |
// Get the local coordinates trasnlated from | |
// the "global" event coordinates. | |
var localCoordinates = targetLocal.globalToLocal( | |
event.pageX, | |
event.pageY | |
); | |
// Set the text of the local element. When | |
// setting the actual position, adjust for | |
// the border of the container. | |
local | |
.text( | |
localCoordinates.x + | |
" / " + | |
localCoordinates.y | |
) | |
.css({ | |
left: (localCoordinates.x - 20 + "px"), | |
top: (localCoordinates.y - 20 + "px") | |
}) | |
.show() | |
; | |
// -------------------------------------- // | |
// -------------------------------------- // | |
// Using the local elements, let's set the | |
// position of the global div relative to the | |
// other target element. | |
var globalCoordinates = targetGlobal.localToGlobal( | |
localCoordinates.x, | |
localCoordinates.y | |
); | |
// Set the text and position the element. | |
// | |
// NOTE: When going from local to global, we | |
// don't need to take into account the local | |
// border when positioning the element since | |
// the element is NOT INSIDE the target | |
// container / context. | |
global | |
.text( | |
globalCoordinates.x + | |
" / " + | |
globalCoordinates.y | |
) | |
.css({ | |
left: (globalCoordinates.x + "px"), | |
top: (globalCoordinates.y + "px") | |
}) | |
.show() | |
; | |
} | |
); | |
}); | |
</script> | |
</head> | |
<body> | |
<h1> | |
Translating Global jQuery Event Coordinates | |
</h1> | |
<div id="target-local"> | |
Local Target | |
</div> | |
<div id="target-global"> | |
Global Target | |
</div> | |
<div id="local"> | |
<!-- This will be populated later. --> | |
</div> | |
<div id="global"> | |
<!-- This will be populated later. --> | |
</div> | |
</body> | |
</html> |
As you can see in the code, I have two local context containers. Then, I have two positioned Div elements. The first div element goes inside the first local context (for local positioning). The second div element stays in the global context, but will positioned globally, relative to the second local context container. When I click in the first container, I translate the global coordinates to the local context to position the first div. When I do this, I have to take into account the border of the local context. Then, I take those local coordinates and I re-translate them back to the global coordinate system, this time in the context of the second container. These new, global coordinates are then used to position the second div.
When I click in first container, I get a page that looks like this:
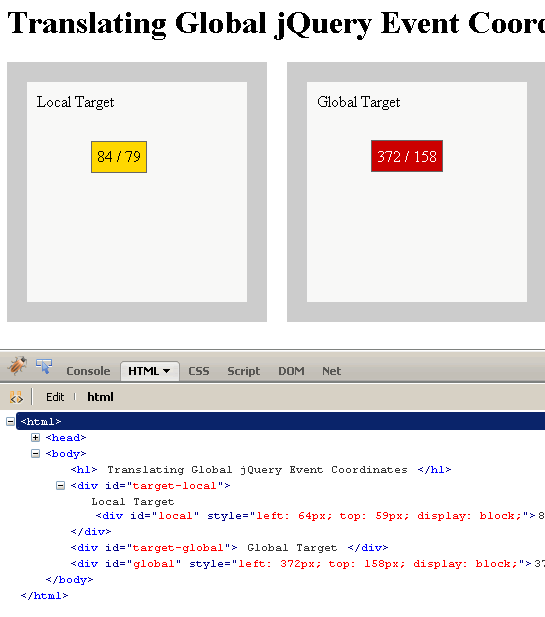
As you can see, I am able to replicate the same visual offset of each positioned element by translating the global mouse event coordinates in and out of different contexts. The reason I have to take the border into account in the first context is because the child element is positioned relative to inner portion of the container, which doesn't include the border width.
This exploration of mouse-event coordinate translation in jQuery covers the most common use cases, in my experience. I am not sure how the offset() method will work if you start dealing with elements that have (or are contained within ancestors that have) "hidden" or "scrolling" overflow values. I assume that such a condition would greatly up the level of complexity in calculating the local coordinate system. But, like I said, I have found that this approach works in most use cases.
Want to use code from this post? Check out the license.
Reader Comments
Ben, great article.. I like the presentation, and ease of using the example. Will explore your blog further.. I have one question, bit off topic.. which software/tool did u use to draw the image which sows the coordinates (www.bennadel.com/resources/uploads/translating_jquery_event_coordinates_locally.gif) .. It is so clean and beautiful, cant wait to know it... thanks again.
bsr.
@BSR,
Thanks! I use Adobe Fireworks for all of my graphics needs. It's like Photoshop, but designed and optimized for web development. It's the perfect merger of bitmap and vector-based graphics.