Using jQuery To Detect CSS-Based Table Display Capabilities
Yesterday, I started to play around with CSS-based table displays for my "Best of ColdFusion 9" contest entry. Based on some very brief testing, it appears, as expected, that Internet Explorer (IE) is the only browser that I have that doesn't support CSS-based table displays. This is something that I might ordinarily deal with via browser-sniffing; but, I believe with the future releases of jQuery, the jQuery team wants us to move away from browser-sniffing and move more towards capability-sniffing.
The difference between browser-sniffing and capability-sniffing is that capability-sniffing checks for individual pieces of functionality rather than entire sets of functionality. The benefit to this approach is that it allows for browsers to be partial upgraded (adding support for some functionality) without breaking the code. With this approach in mind, I wondered if I could programmatically detect CSS-based table display support.
While this was not extensively tested (I only have a few different browsers), it appears that you can detect support for CSS-based table displays by gathering the markup of elements that contain inline table CSS and checking it for the inline CSS; the theory here is that non-supporting browsers will strip out the unsupported table-CSS:
<!DOCTYPE HTML> | |
<html> | |
<head> | |
<title> | |
Detecting CSS-Based Table Display Support With jQuery | |
</title> | |
<style type="text/css"> | |
div.table { | |
display: table ; | |
width: 100% ; | |
} | |
div.table-row { | |
display: table-row ; | |
} | |
div.table-cell { | |
display: table-cell ; | |
} | |
div.first-table-cell { | |
background-color: #E0E0E0 ; | |
width: 50% ; | |
} | |
div.last-table-cell { | |
background-color: #FFE0E0 ; | |
width: 50% ; | |
} | |
/* -- Fixes for non-Table support. -- */ | |
div.table-fix { | |
display: block ; | |
} | |
div.table-fix div.table-row { | |
display: block ; | |
} | |
div.table-fix div.table-row:after { content: "."; display: block; height: 0; clear: both; visibility: hidden; } | |
div.table-fix div.table-row { display: inline-block; } | |
/* required comment for clearfix to work in Opera \*/ | |
* html div.table-fix div.table-row { height:1%; } | |
div.table-fix div.table-row { display:block; } | |
div.table-fix div.table-cell { | |
display: block ; | |
float: left ; | |
} | |
</style> | |
<script type="text/javascript" src="jquery-1.3.2.js"></script> | |
<script type="text/javascript"> | |
// Check to see if this browser supports CSS-based Table | |
// layout displays. | |
var supportsCSSTableLayout = (function( $ ){ | |
// Create nodes with the table-based display values. | |
var tableLayoutTest = $( | |
"<div>" + | |
"<div style=\"display:table;\"></div>" + | |
"<div style=\"display:table-row;\"></div>" + | |
"<div style=\"display:table-cell;\"></div>" + | |
"</div>" | |
); | |
// Get the HTML for the above node and gather all | |
// "table" type displays from the markup (IE browsers | |
// will strip that out of the markup if they do not | |
// support it. | |
var tableMatches = tableLayoutTest.html().match( | |
new RegExp( | |
"table(-(row|cell))?", | |
"gi" | |
) | |
); | |
// Return true if all three table-based displays were | |
// found in the markup. | |
return( | |
(tableMatches && (tableMatches.length == 3)) ? | |
true : false | |
); | |
})( jQuery ); | |
// -------------------------------------------------- // | |
// -------------------------------------------------- // | |
// When the DOM is ready to be interacted with, init. | |
jQuery(function( $ ){ | |
// Output whether or not CSS table display is supported. | |
$( "#has_support" ).text( supportsCSSTableLayout ); | |
// If there is no CSS-based table display support, | |
// then add the fix to the table elements. | |
if (!supportsCSSTableLayout){ | |
$( ".table" ).addClass( "table-fix" ); | |
} | |
}); | |
</script> | |
</head> | |
<body> | |
<h1> | |
Detecting CSS-Based Table Display Support With jQuery | |
</h1> | |
<p> | |
Supports CSS Table Display: | |
<strong id="has_support">...</strong> | |
</p> | |
<div class="table"> | |
<div class="table-row"> | |
<div class="table-cell first-table-cell"> | |
Cell 1 | |
<br /> | |
</div> | |
<div class="table-cell last-table-cell"> | |
Cell 2 | |
<!--- Add breaks here to grow cell. ---> | |
<br /><br /><br /><br /><br /><br /> | |
</div> | |
</div> | |
</div> | |
</body> | |
</html> |
As you can see in the above code, I am creating three DIV elements, each with a different table-display property. I then grab the markup of those DIV elements and see if I can extract all three table-display properties. If I can, I am assuming that table-display is supported.
Here's me running the above code in FireFox (or Safari, or Chrome), which supports CSS-based table display:
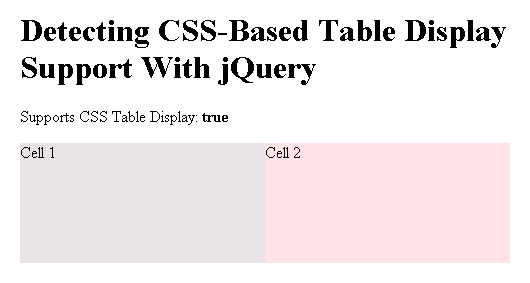
And, here's me running the above code in Internet Explorer 7, which does not support CSS-based table display:
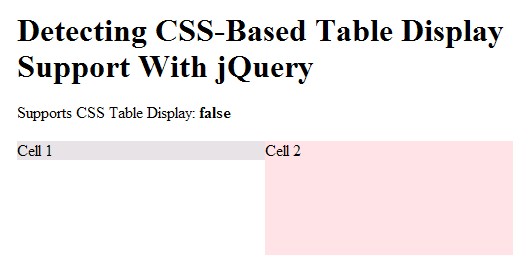
As you can see, the support for CSS-based table display was detected effectively. But again, I have not tested this exhaustively; however, I believe this is a similar approach to feature-detection that the future releases of jQuery will be using.
Want to use code from this post? Check out the license.
Reader Comments
why not use this?
if($.browser.msie && $.browser.version >= 8 ){ // do your thing }
I know they say that it isn't for browser "detection" but seems to work great...
@Steven,
From what I heard John Resig explain at jQuery Conf. 2009, they are moving away from checking for things like "MSIE" and version number because then if MSIE releases a new browser or someone releases some plugin that updates functionality, then your code might not function properly.
For example, I think there's now a way to use the Chrome rendering engine inside of IE (http://code.google.com/chrome/chromeframe/). I haven't looked too much into that, but how does it change the user agent? I am not sure if it does, or if it modifies it in a way that looks like IE. In such a case, you might think you are in a crippled browser, but you are actually in a standards-compliant context. This is where checking individual functionality would be great since it is not browser-specific.
Yah, the idea of moving away from browser sniffing pre-dates jQuery.
Back in the day it was relatively easy to check for MSIE or Netscape. Nowadays you'd have to check for MSIE, Firefox, Chrome, Opera, etc.
Not only that, but you'd have to check versions of each.
And when the next cool browser comes out, you need to go back and update all your code O_O
Checking for specific functionality allows your code to continue working in spite of what new browsers, or new browser versions, are introduced.
I think one of the earliest implementations of this was doing "if (document.images)" to see if the browser supported the ability to reference images using that syntax in order to do image-swapping :)
you could kill the regex with this one liner and it would execute a whole lot faster:
return(tableLayoutTest.html().length > 37); // length of the divs if they are empty
@Charlie,
Great explanation! Yeah, I remember doing the if (document.all) check for IE-based stuff back in the day. Oooh, and remember, if (document.layers) for the old netscape stuff! Grrr :)
@Steven,
Oh, I didn't think of that.
nice. thx.
i have a questions, is it possible to split images with the table display css function?
Can I include an image in the tables?
Do you know what I mean?
nice. thx.
i have a questions, is it possible to split images with the table display css function?
Can I include an image in the tables?
Do you know what I mean?
edit:
i mean to post the whole image, but it shall to hulk up a part of the image.
__________
| | image
----------
and of this image I want to want a specific part, which I want to choose.
@Carina,
I am not sure I fully understand; but, you should be able to wrap the image in a fixed-height/width DIV with no overflow and give the image some negative margins.
It sounds like you want to create a clipping of the original image?
ah okay, thx.
Yes, thats right i meant to create a clipping of the original image. Sry for cant explain what ive soght ;-)
Is it heavy to create it?
@Carina,
It would be something like this:
<div style="width: 100px ; height: 100px ; overflow: hidden ;">
<img style="position: relative ; top: -50px ; left: -25px ;" />
</div>
Maybe something like that? Where the top/left position of the IMG will create the clipping in the 100x100 DIV container.
Good Morning Ben Nadel,
yes thx that was what I ve sought!
Omg! That took some doing ;-)
I have also tried with css rect, you know the rect order?
@Carina,
I've seen the CSS-based rect and clip stuff, but I have not played around with it myself. I am not sure how widely supported it is.
This is really useful. Thanks Ben.
@Charlie Griefer:
Yes, I agree about capability sniffing predating jQuery. In fact, Danny Goodman was one of the early proponents of capability sniffing. It's in his JavaScript Bible, which came out LONG ago. Danny Goodman has been with JavaScript since it was HyperScript in Apple's HyperCard application.
@Ben:
What a great capabilities-based way to detect display:table, etc, support!! Well done!!
Unfortunately, I can't use it. I work at a US Federal Government agency, and my pages have to be accessible with JavaScript off. I thought you'd be interested in how I got around that problem.
First of all, we're only required to support 2 major versions back of the top browsers. That means that only MSIE is a problem. Everyone else supports display:table, etc, and has for quite a while.
So I have 2 css files, one of which defines classes that use table, table-row and table-cell, and the other of which defines the same class names, but using the clearfix technique described at http://www.positioniseverything.net/easyclearing.html Then, in the HTML, I use the conditional comment technique, described in http://www.positioniseverything.net/articles/cc-plus.html
Example pseudocode using parens instead of less-than and greater-than:
(link standardscompliantbrowsers.css)
(!--[if lt IE 8])
(link msie6and7.css)
(![endif]--)
In MSIE 6 and 7, I experience the same irregular box heights and widths that you did above. Those aren't usually a problem as long as there isn't a border or background to call attention to their actual shapes. I was considering writing an MSIE-6-and-7-only jQuery loop that would, for each row, set each cell's height to the max height within that row. It's also possible to do the same for widths. In fact, it takes only 2 passes, one to calculate max heights by row and at the same time calculate max widths by column, and another pass to set each cell's height and width to what they should be, based on row and column.
I'm allowed to do that with JavaScript because it's cosmetic. It doesn't affect functionality. The sticky wicket is nesting. My developers are going to want to do the equivalent of tables within tables, so you have to do it innermost up the DOM tree to outermost. What a frickin' pain. jQuery .each() loops use "for ... in" syntax, so there's no easy reverse order mechanism. For now, I'm telling my developers not to divulge the cell sizes with backgrounds or borders.
P.S.: The clearfix technique messes up if you add the code the PIE folks recommend for MSIE for the Mac. It works much better without it. Fortunately, MSIE for the Mac is long dead, so we're not required to support it. Just thought you should know not to include that code, if you want to try it.
@Steve,
Sounds like you are using a pretty good, happy -medium approach. Having to accommodate such a wide range of "experience conditions" can certainly be a huge hassle. Keep on rocking it my man.
@Ben: Thanks. You too my man.
Since you apparently LOVE to experiment with CSS-related stuff, have a look at this, announced just yesterday on Slashdot: http://developers.slashdot.org/story/10/07/17/1645207/Adding-CSS3-Support-To-IE-6-7-and-8-With-CSS3-Pie
Unfortunately, it introduces a TLA collision (three letter acronym). In my previous post here, PIE meant positioniseverything.net. In this new open source project, PIE means Progressive Internet Explorer.
In the comments of the Slashdot article, I posted a comment on the idea of supporting display:table in Internet Explorer via the behavior property and an HTC file. Seems like css3pie.htc would be a great place to put such support, or maybe a new css2pie.htc file. The looming problem I foresee is that it's not a new property, but rather a new value for an existing property (display). You wouldn't want to add it if it meant having to re-implement all of the display property in JavaScript.
Maybe Olivia Munn will read this thread for all of its PIE acronyms.
@Steve,
That CSSPie thing looks very interesting! I'll look further into it; but, in the comments of the SlashDot article, it seems that some people are saying there are some noticeable performance hits. Of course, I'd have to see that for myself. Regardless, thanks for the cool link.
I wonder if you have to add the HTC file to every element of if you can just inherit from the body element or something... of course that might hit performance even more.