Getting IFRAME Window (And Then Document) References With contentWindow
Perviously, when I had an IFRAME tag on a page and I wanted to get access to the document object within that IFRAME, I would use the top window's "frames" collection:
window.frames[ "my-frame" ].document |
I did this because I could never figure out how to get at the document object directly from the IFRAME reference itself. However, just yesterday, in a comment about my jQuery print() plugin, Todd Rafferty pointed me to an existing print plugin that was able to accomplish this goal. The code, made references to an IFRAME property called "contentWindow". I had never seen this before, but after some Googling, it looks like it was originally an IE property of IFRAMEs that granted access to the "window" of the IFRAME.
Once I have the window object of the IFRAME, I can easily get at the document from it. But, I was nervous that this might be an IE-only property, so I set up the following test using both window-reference methodologies:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | |
<html> | |
<head> | |
<title>Getting IFrame Window Reference With contentWindow</title> | |
<script type="text/javascript" src="jquery-1.3.2.js"></script> | |
<script type="text/javascript"> | |
// When the document has loaded, add an iFrame. | |
$( | |
function(){ | |
var jFrame = $( "<iframe name='my-frame'>" ); | |
var objDoc = null; | |
// Set frame properties and add it to the body. | |
jFrame | |
.css( "width", "400px" ) | |
.css( "height", "100px" ) | |
.appendTo( $( "body" ) ) | |
; | |
// Now, we are going to use two methods for getting | |
// the iFrame window reference and thereby the | |
// document reference such that we can write to it. | |
// Use FRAMES array: | |
objDoc = window.frames[ "my-frame" ].document; | |
objDoc.write( "Gotten via FRAMES <br />" ); | |
// Use the contentWindow property of the iFrame. | |
objDoc = jFrame[ 0 ].contentWindow.document; | |
objDoc.write( "Gotten via contentWindow <br />" ); | |
// Close the document. | |
objDoc.close(); | |
} | |
); | |
</script> | |
</head> | |
<body> | |
<h1> | |
Getting IFrame Window Reference With contentWindow | |
</h1> | |
<p> | |
An iFrame will be added below this: | |
</p> | |
</body> | |
</html> |
When running this code, I got the following output on FireFox, IE (6,7), Safari, and Chrome:
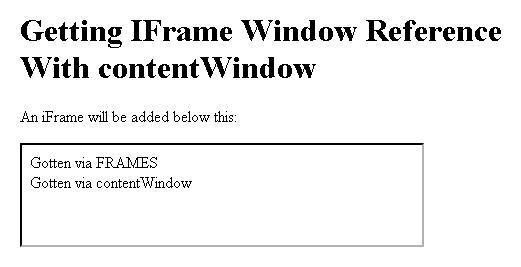
Rock on! It looks like contentWindow returns the window reference of the IFRAME on all relevant browsers. That's an awesome property. Thanks Todd!
Want to use code from this post? Check out the license.
Reader Comments
Ben,
There's also a contentDocument property, but not as widely supported (IE doesn't support it, although it's part of the W3C standard). In the past, I've used something like
var iframeDocument = iframeObject.contentDocument ? iframeObject.contentDocument : iframeObject.contentWindow.document;
Having said that, referencing the frames collection works pretty much everywhere, and as your tests show, I think all the modern browsers implement contentWindow.
@Ryan,
Yeah, I came across contentDocument in my Googling, but as you are saying, it's not widely supported. I think getting the window reference is a nice route as you need the window reference a lot as well.
It would be nice if they all supported contentDocument, but until then :)
@Ben
http://scheduler.cfunited.com/js/scheduler.js
If you search for "getIFrameDocument" that's what I used. It works in basically every browser, new and old. :)
@Elliott,
Looks pretty solid. For funzies, I think you could turn this into short-circuit evaluation:
return(
. . . . iframe.contentDocument ||
. . . . iframe.contentWindow.document ||
. . . . iframe.document
. . . . );
You rock men, i've been looking for this solution for hours ;)
Thank you
Can I use the iframe to fill up forms on another website. For example my task is to open websites with filled up forms. What can I do to do this?
In fact I want to open other websites in iframe and want to use javascript to hold the document inside iframe and to fill up forms inside it.
@Muhammad
You can only interact with the document of an iframe if the document it loaded is on the same domain.
So it would depend on what you meant by "other websites".
Thanks... this was exactly what I was looking for... In jQuery, here is how to get the window document using the approach mentioned here:
$("iframe")[0].contentWindow // == Document Object
Thanks.
HI,
can u please explain me how to read enclosing html document title in the iframe.
e.g
<html>
<title>test</title>
<body>
<iframe src="abc.html">
-- i want the title text here -->
</iframe
@Syam,
Once you have the document object, you should be able to read the title vis the document.title property. So, using your code, you can probably do:
$( "iframe" )[ 0 ].contentWindow.document.title
I hope that helps.
@Ben
I'm trying to use you implementation for another behavior but it only works when I debug the script, appreciate any help.
What I'm trying to do is to catch an event from the iframe to react in the main document. For example: If you click a link on the iframe, there is a JQuery function declared on the main object to catch that using the contentWindow and searching for the object. Like this:
it works...but only when I debug the script and do a watch on the objDoc. Any thoughts?
In case you want know. I already found the problem. I must add the .load() method over the iframe like this:
That way if I click the link in the iframe it reacts in the outside content. Anyway your post help me a lot!
Thanks!!
I am trying to get the TITLE value of an IFRAME in my web page.
Below is the code I have been battling with; please take note of the commented ALERTS, showing all the different ways I have been trying this.
Can anyone please help me get the IFRAME's TITLE string?
var oIframe = document.getElementById('FF05');
var oDoc5 = (oIframe.contentWindow || oIframe.contentDocument || oIframe.contentWindow.document || oIframe.document);
// if (oDoc5.document) oDoc5 = oDoc5.document;
alert("here at minimize_ALL #5 part 4 !!! num = " + num);
//alert("Title 5 1 = " + getIFrameDocument(oIframe).title + " <<<");
//alert("Title 5 2 = " + oIframe.title + " <<<");
alert("Title 5 3 = " + oDoc5.document.title + "<<<");
//alert("Title 5 4 = " + oIframe.contentDocument.title + " <<<");
//alert("Title 5 5 = " + FF05.document.title + " <<<");
if ( FF05.document.title == "Cannot find server")
document.getElementById("FF05").height = 0;
if ( FF05.document.title == "HTTP 404 Not Found")
document.getElementById("FF05").height = 0;
All -
To be more precise, what the above code is looking for is if the page which was attempred to load into the IFRAME was found or not. In the past I used the TITLE, but it appears some new security thingy says we can't get the title from an Iframe for a foreign URL.
Thanks for any help you can give !!!
Hi,
I would like to know whether it is possible to fill contents of a form which is displayed in our page using iframe dynamically. I have seen so many advertising applications doing the same. An eg will be as follows :
Say my website is www.mywebsite.com. and i am using iframe to load another site into my page like this :
[CODE]
<iframe src="http://www.getmeaticket.co.uk/competition/1-000-petrol-voucher?affiliateid=573&campaignid=218" width="90%"
height="90%"></iframe>
[/CODE]
The website over here contains a form and i need to fill it up dynamically using values from my database(details of the user logged in) so that he just need to click on the submit button without actually filling in the form..
Can anyone you tell me how this is possible...
Thanks
Roy M J
@Roy,
Here is what I have discovered... With an Iframe, loaded from a foreign web site, you have no access to what data is in the Iframe, which means you cannot write into it. Something about security in the newest releases of browsers.
I have given up on what I was attempting to do because of this
@Jeffrey,
Hi Jeffrey,
I have succeeded in doing the above process using php. First i get the entire page of the other website into a single variable in php. Then just echo that in another page in our site. and this page in our site will be called in iframe. And we can do anything with the content of the website. :)..
Thanks for the post.
I'm trying to use the same idea, but with one small difference.
1. I need to dynamically create an iframe element and then write into it. I'm using the same technique - getting the document of the iframe and then using document.write().
But, is it possible to access the document object of the iframe before appending it to the body? I keep getting undefined.
2. I noticed that you're not using objDoc.open() before writing. Is that the correct way? I saw other posts where it was different.
Thanks again.
Very good...Its perfect
Hi Ben,
Please can you resolve my issue.
In the below Script, I am writing the Iframe.
We see that, by using this, JS used in the ifrm.document.write(), is appearing inside the Iframe but Openx ads doesn't appear at the front end.
<iframe id="openxi-339762" name="openxi-339762" src="about:blank" frameborder="0" scrolling="no" width="728" height="90"></iframe>
ifrm=document.getElementById("openxi-339762");
ifrm = (ifrm.contentWindow) ? ifrm.contentWindow : (ifrm.contentDocument.document) ? ifrm.contentDocument.document : ifrm.contentDocument;
ifrm.document.open();
ifrm.document.write('<script type="text/javascript">if (!window.OX_ads) { OX_ads = []; } OX_ads.push({ "auid" : "339762" });<\/script><script src="http://us-ads.openx.net/w/1.0/jstag"><\/script>');
ifrm.document.close();
Please suggest how to resolve this in IE browser.